Archive
This post is archived and may contain outdated information. It has been set to 'noindex' and should stop showing up in search results.
This post is archived and may contain outdated information. It has been set to 'noindex' and should stop showing up in search results.
PHP: Using Ternary Operator Condition Checks
Apr 5, 2012ProgrammingComments (0)
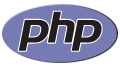
A ternary condition check takes the form:
CONDITION CHECK ? IF_TRUE : IF_FALSE;
The "?" goes after your condition check, and the ":" separates the true or false branches. The false branch is essentially the "else" of a condition check.
Here is a simple condition check using the ubiquitous if/else structure (one of the many ways to write it out):
if ($name == 'Jenkins')
echo 'Hello Jenkins!';
else
echo 'Who are you?';
The same check can be done with the ternary operator like so:
echo $name == 'Jenkins' ? 'Hello Jenkins!' : 'Who are you?';
If concatenating a ternary comparison, put it inside parentheses:
echo 'Hello ' . ($name == 'Jenkins' ? 'Jenkins' : 'Guest') . ', welcome to my site!';
$name = isset($_GET['name']) ? $_GET['name'] : 'Guest';